Materials#
concreteproperties requires material properties to be defined for the concrete and steel components of the reinforced concrete section. Any number of different material properties can be used for a single cross-section. For example, higher strength precast sections can be topped with lower grade in-situ slabs, and high tensile steel can be used in combination with normal grade reinforcing steel.
The structural behaviour of both concrete and steel materials are described by Stress-Strain Profiles.
Note
In concreteproperties, a positive sign is given to compressive forces, stresses and strains, while a negative sign is given to tensile forces, stresses and strains.
Concrete#
- class Concrete(name: str, density: float, stress_strain_profile: ConcreteServiceProfile, ultimate_stress_strain_profile: ConcreteUltimateProfile, alpha_squash: float, flexural_tensile_strength: float, colour: str)[source]
Class for a concrete material.
- Parameters
name (str) – Concrete material name
density (float) – Concrete density (mass per unit volume)
stress_strain_profile (
ConcreteServiceProfile
) – Service concrete stress-strain profileultimate_stress_strain_profile (
ConcreteUltimateProfile
) – Ultimate concrete stress-strain profilealpha_squash (float) – Factor that modifies the concrete compressive strength at squash load
flexural_tensile_strength (float) – Absolute value of the concrete flexural tensile strength
colour (str) – Colour of the material for rendering
Steel#
- class Steel(name: str, density: float, stress_strain_profile: SteelProfile, colour: str)[source]
Class for a steel material.
- Parameters
name (str) – Steel material name
density (float) – Steel density (mass per unit volume)
stress_strain_profile – Ultimate steel stress-strain profile
colour (str) – Colour of the material for rendering
Stress-Strain Profiles#
concreteproperties uses stress-strain profiles to define material behaviour for both
service and ultimate analyses. A Concrete
object
requires both a service stress-strain profile (calculation of area properties,
moment-curvature analysis, elastic and service stress analysis) and an ultimate
stress-strain profile (ultimate bending capacity, moment interaction diagram, biaxial
bending diagram, ultimate stress analysis). A
Steel
object only requires one stress-strain
profile which is used for both service and ultimate analyses.
Note
Stress values are interpolated from stresses and strains supplied to the profile. If the strain is outside of the range of the stress-strain profile, the stress is extrapolated based off the closest two points of the stress-strain profile.
- class StressStrainProfile(strains: List[float], stresses: List[float])[source]
Abstract base class for a material stress-strain profile.
Implements a piecewise linear stress-strain profile. Positive stresses & strains are compression.
- Parameters
strains (List[float]) – List of strains (must be increasing or equal)
stresses (List[float]) – List of stresses
- print_properties(fmt: Optional[str] = '8.6e')[source]
Prints the stress-strain profile properties to the terminal.
- Parameters
fmt (Optional[str]) – Number format
- plot_stress_strain(title: Optional[str] = 'Stress-Strain Profile', **kwargs) matplotlib.axes.Axes [source]
Plots the stress-strain profile.
- Parameters
title (Optional[str]) – Plot title
kwargs – Passed to
plotting_context()
- Returns
Matplotlib axes object
- Return type
Concrete Service Stress-Strain Profiles#
Note
Unless assigned in the class constructor, the elastic_modulus
of the concrete is
determined by the initial compressive slope of the stress-strain profile. This
elastic_modulus
is used in the calculation of area properties and elastic stress
analysis.
Generic Concrete Service Profile#
- class ConcreteServiceProfile(strains: List[float], stresses: List[float], ultimate_strain: float)[source]
Bases:
StressStrainProfile
Abstract class for a concrete service stress-strain profile.
- Parameters
strains (List[float]) – List of strains (must be increasing or equal)
stresses (List[float]) – List of stresses
ultimate_strain (float) – Concrete strain at failure
from concreteproperties.stress_strain_profile import ConcreteServiceProfile
ConcreteServiceProfile(
strains=[-5 / 35e3, -4 / 35e3, -3 / 35e3, 0, 40 / 35e3, 0.003],
stresses=[0, 0, -3, 0, 40, 40],
ultimate_strain=0.003,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
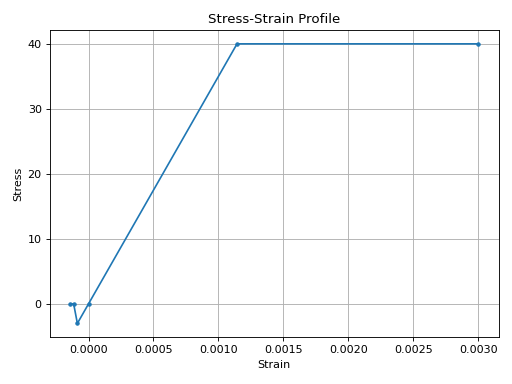
ConcreteServiceProfile Stress-Strain Profile#
Linear Concrete Service Profile#
- class ConcreteLinear(elastic_modulus: float, ultimate_strain: float = 1)[source]
Bases:
ConcreteServiceProfile
Class for a symmetric linear stress-strain profile.
- Parameters
elastic_modulus (float) – Elastic modulus of the stress-strain profile
ultimate_strain (Optional[float]) – Concrete strain at failure
from concreteproperties.stress_strain_profile import ConcreteLinear
ConcreteLinear(elastic_modulus=35e3).plot_stress_strain()
(Source code, png, hires.png, pdf)
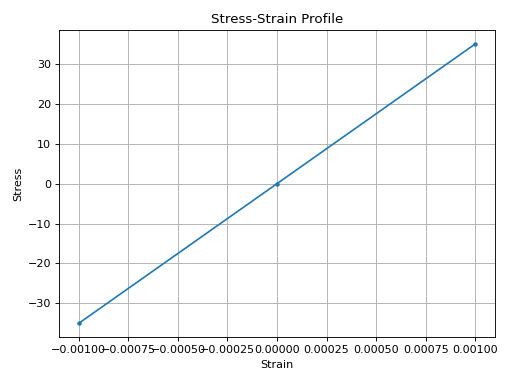
ConcreteLinear Stress-Strain Profile#
Linear Concrete (No Tension) Service Profile#
- class ConcreteLinearNoTension(elastic_modulus: float, ultimate_strain: float = 1)[source]
Bases:
ConcreteLinear
Class for a linear stress-strain profile with no tensile strength.
- Parameters
elastic_modulus (float) – Elastic modulus of the stress-strain profile
ultimate_strain (Optional[float]) – Concrete strain at failure
from concreteproperties.stress_strain_profile import ConcreteLinearNoTension
ConcreteLinearNoTension(elastic_modulus=35e3).plot_stress_strain()
(Source code, png, hires.png, pdf)
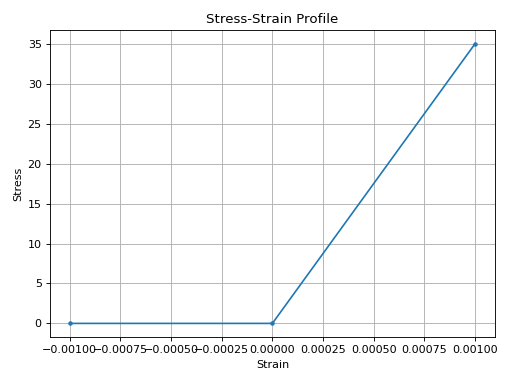
ConcreteLinearNoTension Stress-Strain Profile#
Eurocode Non-Linear Concrete Service Profile#
- class EurocodeNonLinear(elastic_modulus: float, ultimate_strain: float, compressive_strength: float, compressive_strain: float, tensile_strength: float, tension_softening_stiffness: float, n_points_1: Optional[int] = 10, n_points_2: Optional[int] = 3)[source]
Bases:
ConcreteServiceProfile
Class for a non-linear stress-strain relationship to EC2.
Tension is modelled with a symmetric
elastic_modulus
until failure attensile_strength
, after which the tensile stress reduces according to thetension_softening_stiffness
.- Parameters
elastic_modulus (float) – Concrete elastic modulus (\(E_{cm}\))
ultimate_strain (float) – Concrete strain at failure (\(\epsilon_{cu1}\))
compressive_strength (float) – Concrete compressive strength (\(f_{cm}\))
compressive_strain (float) – Strain at which the concrete stress equals the compressive strength (\(\epsilon_{c1}\))
tensile_strength (float) – Concrete tensile strength
tension_softening_stiffness (float) – Slope of the linear tension softening branch
n_points_1 (Optional[int]) – Number of points to discretise the curve prior to the peak stress
n_points_2 (Optional[int]) – Number of points to discretise the curve after the peak stress
from concreteproperties.stress_strain_profile import EurocodeNonLinear
EurocodeNonLinear(
elastic_modulus=35e3,
ultimate_strain=0.0035,
compressive_strength=40,
compressive_strain=0.0023,
tensile_strength=3.5,
tension_softening_stiffness=7e3,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
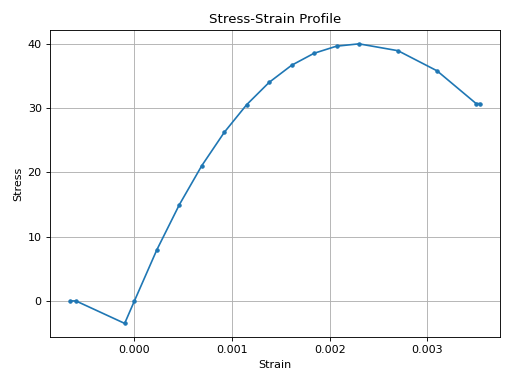
EurocodeNonLinear Stress-Strain Profile#
Concrete Ultimate Stress-Strain Profiles#
Note
Unless assigned in the class constructor, the ultimate_strain
of the concrete is
taken as the largest compressive strain in the stress-strain profile. This
ultimate_strain
defines the curvature and strain profile used in ultimate
analyses.
Warning
concreteproperties currently only supports a single unique ultimate_strain
to be
used for a given ConcreteSection
. While
multiple concrete materials, with differing stress-strain profiles, can be
used within a given ConcreteSection
, the
ultimate analysis will use the smallest value of the ultimate_strain
amongst the
various concrete materials to define the strain profile at ultimate.
Generic Concrete Ultimate Profile#
- class ConcreteUltimateProfile(strains: List[float], stresses: List[float], compressive_strength: float)[source]
Bases:
StressStrainProfile
Abstract class for a concrete ultimate stress-strain profile.
- Parameters
strains (List[float]) – List of strains (must be increasing or equal)
stresses (List[float]) – List of stresses
compressive_strength (float) – Concrete compressive strength
from concreteproperties.stress_strain_profile import ConcreteUltimateProfile
ConcreteUltimateProfile(
strains=[-20 / 30e3, 0, 20 / 30e3, 30 / 25e3, 40 / 20e3, 0.003],
stresses=[0, 0, 20, 30, 40, 40],
compressive_strength=32,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
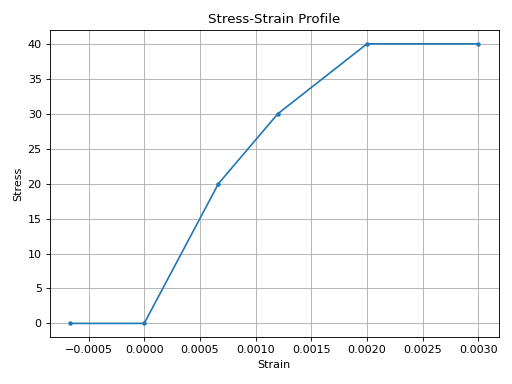
ConcreteUltimateProfile Stress-Strain Profile#
Rectangular Stress Block#
- class RectangularStressBlock(compressive_strength: float, alpha: float, gamma: float, ultimate_strain: float)[source]
Bases:
ConcreteUltimateProfile
Class for a rectangular stress block.
- Parameters
compressive_strength (float) – Concrete compressive strength
alpha (float) – Factor that modifies the concrete compressive strength
gamma (float) – Factor that modifies the depth of the stress block
ultimate_strain (float) – Concrete strain at failure
from concreteproperties.stress_strain_profile import RectangularStressBlock
RectangularStressBlock(
compressive_strength=40,
alpha=0.85,
gamma=0.77,
ultimate_strain=0.003,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
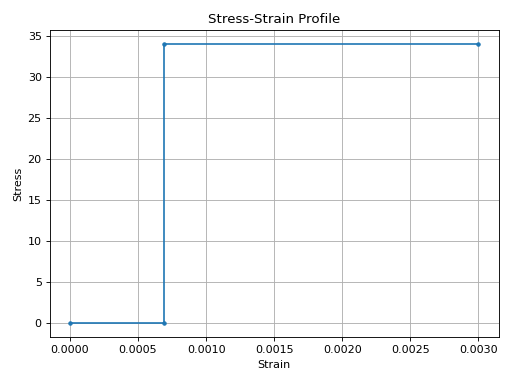
RectangularStressBlock Stress-Strain Profile#
Bilinear Ultimate Profile#
- class BilinearStressStrain(compressive_strength: float, compressive_strain: float, ultimate_strain: float)[source]
Bases:
ConcreteUltimateProfile
Class for a bilinear stress-strain relationship.
- Parameters
compressive_strength (float) – Concrete compressive strength
compressive_strain (float) – Strain at which the concrete stress equals the compressive strength
ultimate_strain (float) – Concrete strain at failure
from concreteproperties.stress_strain_profile import BilinearStressStrain
BilinearStressStrain(
compressive_strength=40,
compressive_strain=0.00175,
ultimate_strain=0.0035,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
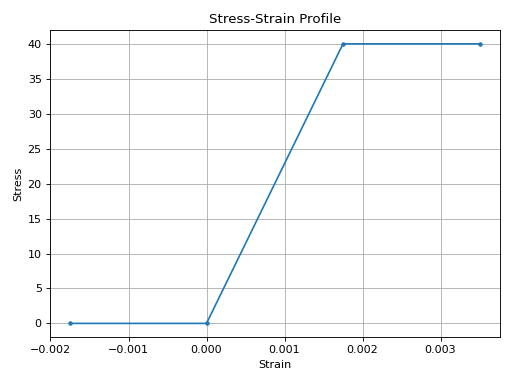
BilinearStressStrain Stress-Strain Profile#
Eurocode Parabolic Ultimate Profile#
- class EurocodeParabolicUltimate(compressive_strength: float, compressive_strain: float, ultimate_strain: float, n: float, n_points: Optional[int] = 10)[source]
Bases:
ConcreteUltimateProfile
Class for an ultimate parabolic stress-strain relationship to EC2.
- Parameters
compressive_strength (float) – Concrete compressive strength
compressive_strain (float) – Strain at which the concrete stress equals the compressive strength
ultimate_strain (float) – Concrete strain at failure
n (float) – Parabolic curve exponent
n_points (Optional[int]) – Number of points to discretise the parabolic segment of the curve
from concreteproperties.stress_strain_profile import EurocodeParabolicUltimate
EurocodeParabolicUltimate(
compressive_strength=40,
compressive_strain=0.00175,
ultimate_strain=0.0035,
n=2,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
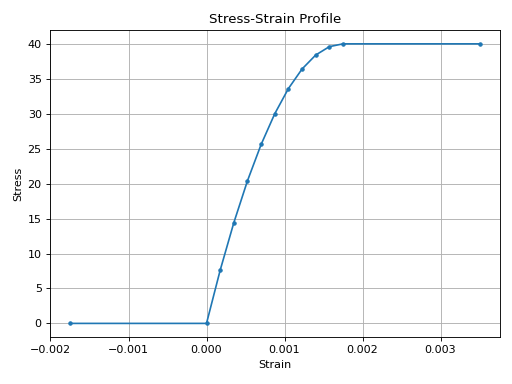
EurocodeParabolicUltimate Stress-Strain Profile#
Steel Stress-Strain Profiles#
Generic Steel Profile#
- class SteelProfile(strains: List[float], stresses: List[float], yield_strength: float, elastic_modulus: float, fracture_strain: float)[source]
Bases:
StressStrainProfile
Abstract class for a steel stress-strain profile.
- Parameters
strains (List[float]) – List of strains (must be increasing or equal)
stresses (List[float]) – List of stresses
yield_strength (float) – Steel yield strength
elastic_modulus (float) – Steel elastic modulus
fracture_strain (float) – Steel fracture strain
from concreteproperties.stress_strain_profile import SteelProfile
SteelProfile(
strains=[-0.05, -0.03, -0.02, -500 / 200e3, 0, 500 / 200e3, 0.02, 0.03, 0.05],
stresses=[-600, -600, -500, -500, 0, 500, 500, 600, 600],
yield_strength=500,
elastic_modulus=200e3,
fracture_strain=0.05,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
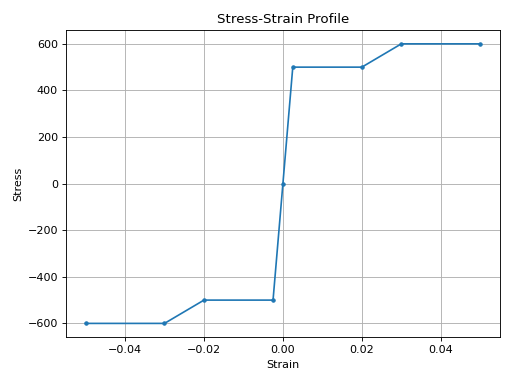
SteelProfile Stress-Strain Profile#
Elastic-Plastic Steel Profile#
- class SteelElasticPlastic(yield_strength: float, elastic_modulus: float, fracture_strain: float)[source]
Bases:
SteelProfile
Class for a perfectly elastic-plastic steel stress-strain profile.
- Parameters
yield_strength (float) – Steel yield strength
elastic_modulus (float) – Steel elastic modulus
fracture_strain (float) – Steel fracture strain
from concreteproperties.stress_strain_profile import SteelElasticPlastic
SteelElasticPlastic(
yield_strength=500,
elastic_modulus=200e3,
fracture_strain=0.05,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
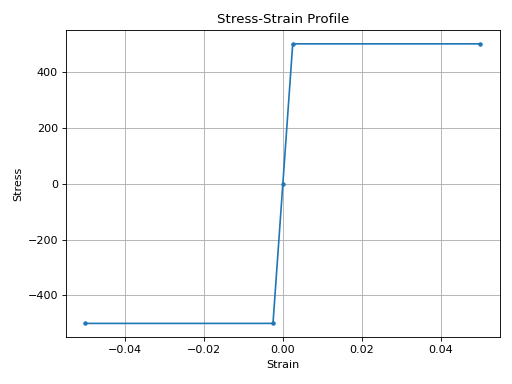
SteelElasticPlastic Stress-Strain Profile#
Elastic-Plastic Hardening Steel Profile#
- class SteelHardening(yield_strength: float, elastic_modulus: float, fracture_strain: float, ultimate_strength: float)[source]
Bases:
SteelProfile
Class for a steel stress-strain profile with strain hardening.
- Parameters
yield_strength (float) – Steel yield strength
elastic_modulus (float) – Steel elastic modulus
fracture_strain (float) – Steel fracture strain
ultimate_strength (float) – Steel ultimate strength
from concreteproperties.stress_strain_profile import SteelHardening
SteelHardening(
yield_strength=500,
elastic_modulus=200e3,
fracture_strain=0.05,
ultimate_strength=600,
).plot_stress_strain()
(Source code, png, hires.png, pdf)
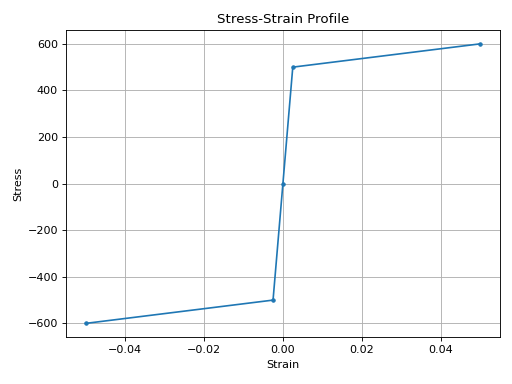
SteelHardening Stress-Strain Profile#