6.1. AS 3600:2018#
- class AS3600[source]
Design code class for Australian standard AS 3600:2018.
Note
Note that this design code only supports
Concrete
andSteelBar
material objects. MeshedSteel
material objects are not supported as this falls under the composite structures design code.Inits the AS3600 class.
Using the AS 3600:2018 design code starts by creating an
AS3600
object:
from concreteproperties.design_codes.as3600 import AS3600
design_code = AS3600()
After a ConcreteSection
object has been
created it must be assigned to the design code:
design_code.assign_concrete_section(concrete_section=concrete_section)
- AS3600.assign_concrete_section(concrete_section)[source]
Assigns a concrete section to the design code.
- Parameters
concrete_section (
ConcreteSection
) – Concrete section object to analyse
Note
To maintain unit consistency, the cross-section dimensions should be entered in [mm].
6.1.1. Creating Material Properties#
The AS3600
class can be used to easily create
material objects whose attributes comply with the standard.
- AS3600.create_concrete_material(compressive_strength, colour='lightgrey')[source]
Returns a concrete material object to AS 3600:2018.
Material assumptions
Density: 2400 kg/m3
Elastic modulus: Interpolated from Table 3.1.2
Service stress-strain profile: Linear with no tension, compressive strength at \(0.9f'_c\)
Ultimate stress-strain profile: Rectangular stress block, parameters from Cl. 8.1.3
Alpha squash: From Cl. 10.6.2.2
Flexural tensile strength: From Cl. 3.1.1.3
- Parameters
compressive_strength (
float
) – Characteristic compressive strength of concrete at 28 days in megapascals (MPa)colour (
str
, default:'lightgrey'
) – Colour of the concrete for rendering
- Raises
ValueError – If
compressive_strength
is not between 20 MPa and 100 MPa.- Returns
Concrete
– Concrete material object
from concreteproperties.design_codes.as3600 import AS3600
design_code = AS3600()
concrete = design_code.create_concrete_material(compressive_strength=40)
concrete.stress_strain_profile.plot_stress_strain(
title=f"{concrete.name} - Service Profile"
)
concrete.ultimate_stress_strain_profile.plot_stress_strain(
title=f"{concrete.name} - Ultimate Profile"
)
- AS3600.create_steel_material(yield_strength=500, ductility_class='N', colour='grey')[source]
Returns a steel bar material object.
Material assumptions
Density: 7850 kg/m3
Elastic modulus: 200000 MPa
Stress-strain profile: Elastic-plastic, fracture strain from Table 3.2.1
- Parameters
yield_strength (
float
, default:500
) – Steel yield strengthductility_class (
str
, default:'N'
) – Steel ductility class (“N” or “L”)colour (
str
, default:'grey'
) – Colour of the steel for rendering
- Raises
ValueError – If
ductility_class
is not “N” or “L”- Returns
SteelBar
– Steel material object
from concreteproperties.design_codes.as3600 import AS3600
design_code = AS3600()
steel = design_code.create_steel_material()
steel.stress_strain_profile.plot_stress_strain(
title=f"{steel.name} - Stress-Strain Profile"
)
(Source code
, png
, hires.png
, pdf
)
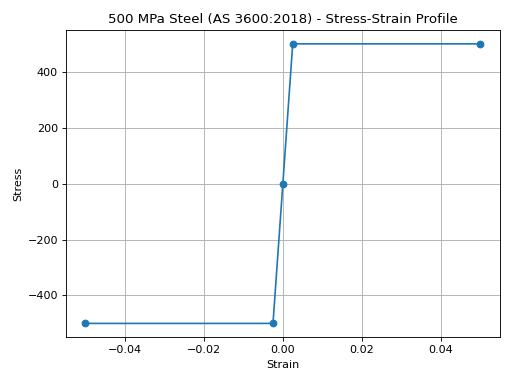
6.1.2. Calculating Section Properties#
Analysis methods can be called from the
AS3600
object similar to
ConcreteSection
object. The following
methods are identical to those found in the
ConcreteSection
object, i.e. do not apply
any capacity reduction factors:
The following methods have been modified for AS 3600:2018, with codified capacity reduction factors applied.
- AS3600.ultimate_bending_capacity(theta=0, n_design=0, phi_0=0.6)[source]
Calculates the ultimate bending capacity with capacity factors to AS 3600:2018.
- Parameters
theta (
float
, default:0
) – Angle (in radians) the neutral axis makes with the horizontal axis (\(-\pi \leq \theta \leq \pi\))n_design (
float
, default:0
) – Design axial force, N*phi_0 (
float
, default:0.6
) – Compression dominant capacity reduction factor, see Table 2.2.2(d)
- Returns
Tuple
[UltimateBendingResults
,UltimateBendingResults
,float
] – Factored and unfactored ultimate bending results objects, and capacity reduction factor (factored_results, unfactored_results, phi)
- AS3600.moment_interaction_diagram(theta=0, limits=[('D', 1.0), ('N', 0.0)], control_points=[('fy', 1.0)], labels=None, n_points=24, n_spacing=None, phi_0=0.6, progress_bar=True)[source]
Generates a moment interaction diagram with capacity factors to AS 3600:2018.
See
concreteproperties.concrete_section.ConcreteSection.moment_interaction_diagram()
for allowable control points.Note
When providing
"N"
tolimits
orcontrol_points
,"N"
is taken to be the unfactored net (nominal) axial load \(N^{*} / \phi\).- Parameters
theta (
float
, default:0
) – Angle (in radians) the neutral axis makes with the horizontal axis (\(-\pi \leq \theta \leq \pi\))limits (
List
[Tuple
[str
,float
]], default:[('D', 1.0), ('N', 0.0)]
) – List of control points that define the start and end of the interaction diagram. List length must equal two. The default limits range from concrete decompression strain to the pure bending point.control_points (
List
[Tuple
[str
,float
]], default:[('fy', 1.0)]
) – List of additional control points to add to the moment interaction diagram. The default control points include the balanced point (fy=1
). Control points may lie outside the limits of the moment interaction diagram as long as equilibrium can be found.labels (
Optional
[List
[str
]], default:None
) – List of labels to apply to thelimits
andcontrol_points
for plotting purposes. The first two values inlabels
apply labels to thelimits
, the remaining values apply labels to thecontrol_points
. If a single value is provided, this value will be applied to bothlimits
and allcontrol_points
. The length oflabels
must equal1
or2 + len(control_points)
.n_points (
int
, default:24
) – Number of points to compute including and between thelimits
of the moment interaction diagram. Generates equally spaced neutral axes between thelimits
.n_spacing (
Optional
[int
], default:None
) – If provided, overridesn_points
and generates the moment interaction diagram usingn_spacing
equally spaced axial loads. Note that usingn_spacing
negatively affects performance, as the neutral axis depth must first be located for each point on the moment interaction diagram.phi_0 (
float
, default:0.6
) – Compression dominant capacity reduction factor, see Table 2.2.2(d)progress_bar (
bool
, default:True
) – If set to True, displays the progress bar
- Returns
Tuple
[MomentInteractionResults
,MomentInteractionResults
,List
[float
]] – Factored and unfactored moment interaction results objects, and list of capacity reduction factors (factored_results, unfactored_results, phis)
- AS3600.biaxial_bending_diagram(n_design=0, n_points=48, phi_0=0.6, progress_bar=True)[source]
Generates a biaxial bending with capacity factors to AS 3600:2018.
- Parameters
n_design (
float
, default:0
) – Design axial force, N*n_points (
int
, default:48
) – Number of calculation pointsphi_0 (
float
, default:0.6
) – Compression dominant capacity reduction factor, see Table 2.2.2(d)progress_bar (
bool
, default:True
) – If set to True, displays the progress bar
- Returns
Tuple
[BiaxialBendingResults
,List
[float
]] – Factored biaxial bending results object and list of capacity reduction factors (factored_results, phis)
See also
For an application of the use of the design code object, see the example AS3600 Design Code.